webpack-file-manager-plugin v 2.0.5
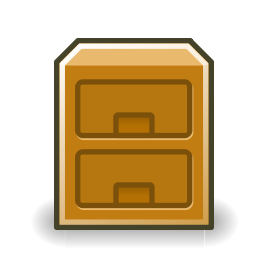
这个 Webpack 插件允许您在构建前或构建后复制、归档(.zip/.tar/.tar.gz)、移动、删除文件和目录。
安装
npm install filemanager-webpack-plugin --save-dev
1
使用
webpack.config.js
const FileManagerPlugin = require('filemanager-webpack-plugin');
module.exports = {
// ...
// ...
plugins: [
new FileManagerPlugin({
onEnd: {
copy: [
{ source: '/path/from', destination: '/path/to' },
{ source: '/path/**/*.js', destination: '/path' },
{ source: '/path/fromfile.txt', destination: '/path/tofile.txt' },
{ source: '/path/**/*.{html,js}', destination: '/path/to' },
{ source: '/path/{file1,file2}.js', destination: '/path/to' },
{ source: '/path/file-[hash].js', destination: '/path/to' }
],
move: [
{ source: '/path/from', destination: '/path/to' },
{ source: '/path/fromfile.txt', destination: '/path/tofile.txt' }
],
delete: [
'/path/to/file.txt',
'/path/to/directory/'
],
mkdir: [
'/path/to/directory/',
'/another/directory/'
],
archive: [
{ source: '/path/from', destination: '/path/to.zip' },
{ source: '/path/**/*.js', destination: '/path/to.zip' },
{ source: '/path/fromfile.txt', destination: '/path/to.zip' },
{ source: '/path/fromfile.txt', destination: '/path/to.zip', format: 'tar' },
{
source: '/path/fromfile.txt',
destination: '/path/to.tar.gz',
format: 'tar',
options: {
gzip: true,
gzipOptions: {
level: 1
},
globOptions: {
nomount: true
}
}
}
]
}
})
],
// ...
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
如果您想要按顺序运行操作,可以将onStart
和onEnd
事件设置为数组。在下面的例子里,在onEnd
事件中,将首先运行copy
操作:
const FileManagerPlugin = require('filemanager-webpack-plugin');
module.exports = {
// ...
// ...
plugins: [
new FileManagerPlugin({
onEnd: [
{
copy: [
{ source: "./dist/bundle.js", destination: "./newfile.js" }
]
},
{
delete: [
"./dist/bundle.js"
]
}
]
})
],
...
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
配置项
new FileManagerPlugin(fileEvents, options)
1
事件
onStart
: 在 webpack 开始打包流程之前要执行的命令。onEnd
: 在 webpack 打包流程结束之后要执行的命令。
名称 | 描述 | 示例 |
---|---|---|
copy | 将单个文件或整个目录从源文件夹复制到目标文件夹。支持通配符。 | copy: [{ source: 'dist/bundle.js', destination: '/home/web/js/'}] |
delete | 删除单个文件或整个目录。 | delete: ['file.txt', '/path/to'] |
move | 移动单个文件或整个目录。 | move: [{ source: 'dist/bundle.js', destination: '/home/web/js/'}] |
mkdir | 创建目录的路径。 | mkdir: [ '/path/to/directory/', '/another/path/' ] |
archive | 归档单个文件或整个目录。默认为.zip ,可以设置format 和options 来修改。使用了node-archiver | archive: [{ source: 'dist/bundle.js', destination: '/home/web/archive.zip' format: 'tar' or 'zip' options: { }}] |